Webcam in C#: AForge.NET March 21, 2009
Posted by haryoktav in Taipei.trackback
AForge.NET is another C# framework to do image processing and others. For further information just go to http://www.aforgenet.com/framework/
Here, I want to show you how to access webcam using AForge libraries. I found that these libraries are more complete and so far work for me.
I can select one of webcams attached in my notebook (a notebook usually has a webcam and I connected another USB webcam). And wow, awesome!
Now, download and extract the AForge libraries from http://www.aforgenet.com/framework/downloads.html, download this project here (using VS2005, don’t forget to change the extension from “.doc’ to “.rar”).. and I hope that you will have fun with your webcam(s).
Also, remember to Add Reference for AForge.Video.dll and AForge.Video.DirectShow.dll into the project.
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Text; using System.Windows.Forms; using AForge.Video; using AForge.Video.DirectShow; namespace cam_aforge1 { public partial class Form1 : Form { private bool DeviceExist = false; private FilterInfoCollection videoDevices; private VideoCaptureDevice videoSource = null; public Form1() { InitializeComponent(); } // get the devices name private void getCamList() { try { videoDevices = new FilterInfoCollection(FilterCategory.VideoInputDevice); comboBox1.Items.Clear(); if (videoDevices.Count == 0) throw new ApplicationException(); DeviceExist = true; foreach (FilterInfo device in videoDevices) { comboBox1.Items.Add(device.Name); } comboBox1.SelectedIndex = 0; //make dafault to first cam } catch (ApplicationException) { DeviceExist = false; comboBox1.Items.Add("No capture device on your system"); } } //refresh button private void rfsh_Click(object sender, EventArgs e) { getCamList(); } //toggle start and stop button private void start_Click(object sender, EventArgs e) { if (start.Text == "&Start") { if (DeviceExist) { videoSource = new VideoCaptureDevice(videoDevices[comboBox1.SelectedIndex].MonikerString); videoSource.NewFrame += new NewFrameEventHandler(video_NewFrame); CloseVideoSource(); videoSource.DesiredFrameSize = new Size(160, 120); //videoSource.DesiredFrameRate = 10; videoSource.Start(); label2.Text = "Device running..."; start.Text = "&Stop"; timer1.Enabled = true; } else { label2.Text = "Error: No Device selected."; } } else { if (videoSource.IsRunning) { timer1.Enabled = false; CloseVideoSource(); label2.Text = "Device stopped."; start.Text = "&Start"; } } } //eventhandler if new frame is ready private void video_NewFrame(object sender, NewFrameEventArgs eventArgs) { Bitmap img = (Bitmap)eventArgs.Frame.Clone(); //do processing here pictureBox1.Image = img; } //close the device safely private void CloseVideoSource() { if (!(videoSource == null)) if (videoSource.IsRunning) { videoSource.SignalToStop(); videoSource = null; } } //get total received frame at 1 second tick private void timer1_Tick(object sender, EventArgs e) { label2.Text = "Device running... " + videoSource.FramesReceived.ToString() + " FPS"; } //prevent sudden close while device is running private void Form1_FormClosed(object sender, FormClosedEventArgs e) { CloseVideoSource(); } } }
Snapshots:
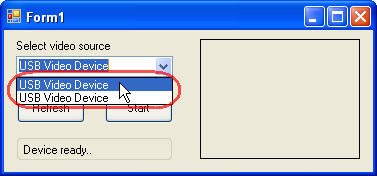
Select webcam source
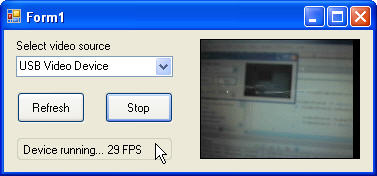
Seeing into the monitor…
how can i get the webcam resolution?
#tiberius
sorry, i haven’t tried yet.. ^^
hello! I have one question! Where can i get text box for camera? I wrote this code in my program, but on the form I nothing saw.
thanks for help
#samo
do you mean the text “Device ready” and “Device running”?
I used a “label” to print those messages, and put it inside a “groupbox” for pretty interface 🙂
this line will change the label: label2.Text = “Device running…”;
you can download the project from the link above
thx.
The Combo Box is empty? or i have to change the text to USB video device
no me devuelve la imagen al picture, creo que el problema esta en
videoSource.NewFrame += new NewFrameEventHandler(video_NewFrame);
Porque puede llegar a ser?
do you have any codes to view the real video of usb web camera in web browser when running your codes? thanks in advance.
Thanks very much.
I’ve tested, It’s works!!
#jason
If there is no USB camera device installed then the combo box will empty
#sebastian
Estoy de acuerdo con usted
pero no tengo ni idea sobre esto
#david
wow, that is beyond of me this time ^^
I ever heard about IP-Camera and we can access it using MJPEG (Motion JPEG). You can search in http://www.codeproject.com how to access MJPEG.
Or using another ways ^^
Ganbatte kudasai !
#AAA
thanks!
Hi… Hary,,
I have make LAN chat application. But how to combine your webcam in my application?
Thanks
Hi…
I’ve making Lan Chat application in C#, but how to add Webcam feature?
Thanks
Hey haryoktav,
Thanks for this post, you saved me a lot of time.
#donny. prazetyo
sorry, that is beyond mine for now.. ^^
#Jay
my pleasure..
can any one plz help ..
i ve a project named: Cursor control through mouse movement in which i have to initiallize webcam n capture stream of images of the user so that i can process that image to find the centre of the user’s eye..
plz help me to initiallize webcam n in taking input stream of images ..
Hi haryoctav,
thank you for the code, never got a webcam picture so fast in a project.
I added some useful code to show the camera property dialog (from the camera driver). Create a new button and the following handler code:
private void props_Click(object sender, EventArgs e)
{
if (videoSource != null)
{
videoSource.DisplayPropertyPage(this.Handle); // modal dialog
//videoSource.DisplayPropertyPage(IntPtr.Zero); // non-modal
}
}
After starting the webcam, this dialog can be shown.
But until now I found no way to get possible resolution and framerate settings from the driver. Any suggestions?
when i use this even though i have the DesiredFrameSize set to 160,120 it is only showing the top left portion of what my webcam is seeing, i cant get it to resize and show all of what my webcam is seeing, any ideas?
hi,
Can anyone help me to use yahoo messenger dll’s in my codings for creating video&audio conferencing using C#.net….if possible please send me links to m.bakhit@yahoo.com
Thank you
Thanks alot man…
This was a real time saver, and the code is also not that hard understand..
Regarding the Video Resolution, its just about keeping the Desired Frame Size according to the picture box size.
I set it to 500 x 400
videoSource.DesiredFrameSize = new Size(500, 400);
And Make the ‘PictureBox’ size bigger in the Design View of your Application. Works Perfectly.
Cheers…………..
How do i save picture which we have captured.Please help.
[…] Aquí les dejo donde aprendí como hacerlo: https://haryoktav.wordpress.com/2009/03/21/webcam-in-c-aforgenet/ […]
Mantab,…
Salam knal mas..^_^
bagus tutorialnya…
tapi saya dapat error di :
if (videoSource.IsRunning) <– NullReferenceExeption was unhandeled
Object reference not set to an instance of an object.
kenapa ya kira2 mas??
#dhoto
thanks man!
#Adit
variable videoSource-nya kosong/null, mustinya sudah dicek oleh baris sebelumnya.. tapi kok tembus ya.. aneh..
#others
sorry for leaving aForge long time ago..
i hope you already have solutions
i face this problem. So how can i solve that?
1. visual studio cannot start debugging because the debug target ‘.exe’ is missing….
2. The name “timer1″does not exist..
now both problem is solve which i mention just now.. But now the combo box never show any device selection (it’s blank). So how can i solve it? please please… 😦
Bang.. saya mau tanya nih.. gimana ya cara biar dapet fps bagus..
Saya set desired frame ratenya (380,437) ngikutin picture boxnya..
cuma dapet 1 fps persecond.. kok kecil banget ya.. ada suggestion ga gimana biar bisa naekin fps nya.. skitar 10++ fps..
Terima Kasih sebelumnya..
setelah di coba2 ini dia “&Start” gak usah pake “&” baru berjalan…^_^
makasih banyak mas…
saya lagi bikin penggerak pointer pake camera dengan menggunakan color filtering (AForge juga)…
sharing2 lagi ya..
lanjutkan….
what muss I do in
//do processing here
???
#Lara
put your image processing algorithms..
can u make a program that put iris cordinates on the screen..??????
please contact @ lucky_xxxxx@yahoo.xxx
#lucky
sorry I am in a busy recently. in fact, i haven’t used AForge already..
thank you for your help
it’s work
Hi we want to save to avi file.
The AviWriter is documented, but it does not exist in AForge. There is no example anywhere of saving to avi file. or any file.
any 1… plz tell me that how can i access ip camera using AForge .net using MJPEG??????
its urgent brothers….. i need it as soon as possible…!
HI,
is there any way to get audio uisng Aforge and stream the captured audio after encoding it….
Pls help if posssible give me some links of examples
Thanks
JIbin
If you are interested in this topic be sure to check out this article: http://www.codeproject.com/KB/miscctrl/webcam_c_sharp.aspx
Hi,
code works for a usb twain webcam but does not work for firewire cam. Is there a solution for that ?
Hi
Using this code, how could I have a still image of the same webcam display in another picturebox?
Hi again….never mind the last post, I sorted it!
Hi Haryoktav!
I have everything working except there is no picture! I know my cam works (using Skype, e.g.), I know the timer works, I get frames rates, etc.
I had the same problem using another aforge example.
Help!
-gf
Please after the download of the framwork AForge.NET where can I put it? How can I execute this code??
Thx, it help me.
hi,this application not work is vs 2010 ide,and nothing warning in the vs 2010 ,you can help me ,i wait your advices as soon as
[…] […]
Can you convert to asp.net i had been converted but no devices found although it working very well as windows App.
Hey haryoktav,
I’m completely new to visual C#. I’ve tried to copy-paste your code into a new windows form project in visual c#. When I run the program, it shows many errors like:
1: The type or namespace name ‘Form1’ could not be found (are you missing a using directive or an assembly reference?)
2: The name ‘comboBox1’ does not exist in the current context
etc.
There are many more suggesting the same for label 2, start, timer1 and picturebox1.
Can you help me with this and suggest how I must proceed from here?
thanks a lot…
i tried out your code..while it shows error
Error 1 The type or namespace name ‘Form1’ could not be found (are you missing a using directive or an assembly reference?) C:\Users\shaji c v\AppData\Local\Temporary Projects\WindowsFormsApplication1\Program.cs 18 33 WindowsFormsApplication1
??.. what do i do??
hOW TO INCLUDE afroge library in c# …..
Hi man,
i tried to convert the code for asp.net. Actually the WebCam was found but the events for example “NewFrame” wasn’t calling.
Have you any ideia, how can i convert this code to asp.net?
Regards,
Andrei Silva
Hello ,,
may my question not here 🙂
I build an application in c# that display a stream of image from the mobile(like webcam) and I want this application appear as an option inside the MSN or Skype
what is the best way
thanks for the help
PLZ anyone can tell me , how to save picture in AFORGER.net
after capturing it . becouse i would like to compare it with next pic .
PLZ do help me
thank you very much,it worked perfectly for me
thanks for your effort and thanks to AForge.Net
I have the problem of not getting any detected devices in the combo box, it remains empty! Debugging the code does’nt give any errors though. Help will be much appreciated. Thanx!
how to select Add Reference and Aforge.video
just download AForge and than place that folder in you app. folder ,than add reference + ………
guys, copy and paste others program is not recommended for a beginner, he just share with us the code but its our initiative to learn from basic, if we copy and paste the program in c# then for sure will have error cause the interface we havent created and the directory willnot be same, we must do fro scratch
Thanks a lot worked just perfect!
i search in google long time just to search how i can save my video capture to directory.. please help me, any sugestioon?? with c# and AForge.net
Respect++ 🙂 A good kick-start for beginners 🙂 Thanks.
I just implemented evrything…..but unable to resolve following error==>
1. NewFrameEventHandler in line :
——————————————————————————–
videoSource.NewFrame += new NewFrameEventHandler(video_NewFrame);
2. NewFrameEventArgs eventArgs in line:
———————————————————————————
private void video_NewFrame(object sender, NewFrameEventArgs eventArgs)
I for all time emailed this webpage post page to all my contacts, because if like to read it after that my links will too.
NewFrameEventArgs – error – you should add references A.Forge.Video…. This solves it forme
when i run this code following error occur please help me in this regards as soon as possible
An unhandled exception of type ‘System.NullReferenceException’ occurred in camera_capture.exe
Additional information: Object reference not set to an instance of an object.
Nice post men, Im was just looking for something like this :). It herlp me a lot
It would be real helpfu if any one if u can solve me these :
1) how can i capture the image from the video using the code above?
2)where will the image be saved and how can i view it? i mean in a folder on my computer or il b required to make a database for the same ?
3)if i need to capture the video currently running and save them every 15 minutes then plz help …
Thanks in advance ..
Hi guys
How can I save to real camera like video ?
obviously like your website however you have to take a look at the spelling
on several of your posts. Several of them are rife with spelling issues and I in finding it
very bothersome to inform the reality nevertheless I’ll surely come
back again.
65. cahit! Hi! I read your question, and it’s possible that this sample help you to solve your problem. You can try the sample here: http://www.camera-sdk.com/p_52-how-to-record-audio-and-video-stream-into-mpeg-4-onvif.html
Besides that you can find more useful information how can you save your camera’s video like a video and how can you take a snapshot.
Thanks alot, it worked well
Hi
Can you please tell me that Is there a way to turn off the flash light of laptop camera using Aforge.Net framwork?
If so then please tell me asap.
Hello ! Great work. I am trying this in VS2008 for Windows CE but it gives me 2 errors in
private void video_NewFrame(object sender, NewFrameEventArgs eventArgs)
{
System.Drawing.Bitmap img = (Bitmap)eventArgs.Frame.Clone();
pictureBox1.Image = img;
}
Error 1 The type ‘System.Drawing.Bitmap’ is defined in an assembly that is not referenced. You must add a reference to assembly ‘System.Drawing, Version=2.0.0.0, Culture=neutral, PublicKeyToken=b03f5f7f11d50a3a’. C:\Users\shayan\Documents\Visual Studio 2008\Projects\WinCE_Webcam_Project\WinCE_Webcam_Project\Form1.cs 65 13 WinCE_Webcam_Project
Error 2 ‘System.Drawing.Bitmap’ does not contain a definition for ‘Clone’ and no extension method ‘Clone’ accepting a first argument of type ‘System.Drawing.Bitmap’ could be found (are you missing a using directive or an assembly reference?) C:\Users\shayan\Documents\Visual Studio 2008\Projects\WinCE_Webcam_Project\WinCE_Webcam_Project\Form1.cs 64 65 WinCE_Webcam_Project
Please tell how to get rid of them ?
+1 for the question on AForge being able to turn webcam LEDs on and off. A bit of searching says this seems to be possible, but only with certain cameras:
http://www.aforgenet.com/framework/docs/html/aed2bddb-f36b-4233-96ac-935e8f66615c.htm
Asalam o alikum, hi hellow can any one help me
i want to just code of webcam using c# or if any have project which is running so please send me at absar_ahmed15@outlook.com but webcam should be autoplay no need to start with button
thanking you,
Thank you very much~!!!
So very easy~!
thank in advance
I do trust all the ideas you’ve offered in your post.
They are very convincing and can certainly work.
Still, the posts are very quick for starters. Could you please extend them a little from subsequent time?
Thanks for the post.
Hello i encountered an error:
An unhandled exception of type System.NotSupportedException occurred in AForge.Video.dll Additional information: No devices of the category
Can anyone help me . thanks